Choosing the Right Java Data Types
There are several reasons for Java having different data types. Java has eight primitive data types:
- byte
- short
- int
- long
- float
- double
- char
- boolean
Of those eight, six are used to represent numbers. Byte, short, int, and long are all used to represent integers, while float and double are used to represent floating-point numbers.
You may be asking, "Why do we need to memorize six different data types just to store numbers?" Well, here are a few reasons:
Size / Performance
Primitive data types store their values directly in the memory location that's allocated for them. As a result, primitive data types have a fixed memory size. An int will always and only take up 4 bytes (32 bits) regardless of its value. The number 7 can be represented in the smallest Java data type, which is a byte (8 bits), or it can be represented as an int which is 4 bytes (32 bits).
- Seven as a byte: 00000111
- Seven as an int: 00000000000000000000000000000111
On most modern computers with hefty amounts of RAM, most programmers might not think they have to worry about how much memory their programs consume. After all, unless you're doing embedded systems programming where memory is limited, storing someone's age as an int rather than byte or short isn't going to cause your program to crash. But if your program is storing or processing a billion people's age, that's a different story. I've heard many stories where developers choose the wrong data type, resulting in unnecessary costs or performance issues for their applications. At scale, consuming four times the necessary memory or storage has real performance implications and can cost your organization millions of dollars. This is the key reason for needing so many data types. Only using what you need means there's less data for the computer to process and less data being stored in memory, files, and databases.
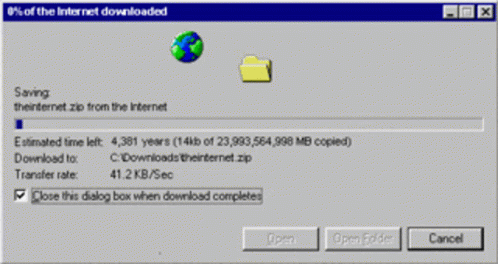
Precision
The other major reason is precision. This is inherently a side effect of saving space by selecting smaller data types. If you use a data type that's smaller, by definition, it can hold less data. This can become an issue when accuracy and precision are important when you don't know ahead of time how large of a value you need to store. According to our textbook, Doubles can hold up to 14 or 15 significant digits, while Float holds up to 6 or 7 significant digits. If you attempt to assign the value 3.1415926535 to a float, you're going to be out of luck. Java will truncate the value to 3.1415927 and your IDE will scream at you. Java gives us multiple data types so that we can select the best type for the data we're storing, but it's up to us as programmers to know what each type can hold and which will work best for our use case.
Type Capacity (Bonus)
Different data types can, of course, hold different maximum and minimum values. But one additional thing to consider is why can a short hold -32,768 to 32,767 rather than -32,768 to 32,768? Since data is stored in binary (which is a base-two number system), it would seem to make sense that the max number would be divisible by two. But that doesn't take into account zero.
Two bytes (the size of a short) contains 16 bits. With signed binary, that means there's a total of 15 bits that can be used to represent numbers (one is used to switch positive/negative). A total of 32,768 values are being stored within those 15 bits, whether positive or negative. However, there's one less positive number because with a positive number, all zeros mean zero; with a negative number, all zeros mean the lowest possible number. Positive numbers have to give up one value to be able to represent zero.
With any length of bits, the maximum number of values that can be represented is always divisible by two, but that doesn't mean what the values represent always will be since zero must be counted as a possible value.
Here's an illustration from my IDE's debugger showing the binary values of each variable: